Co-Founder Taliferro
The Art of API Design: Crafting Interfaces for Seamless Integration
API (Application Programming Interface) design is the backbone of modern software development, facilitating the smooth interaction and integration of diverse software components. An API acts as a conduit, connecting front-end and back-end systems, enabling the seamless sharing of data and functionality between various software applications. A well-crafted API holds the power to elevate the usability and performance of a software system. In this comprehensive essay, we embark on a journey to unravel the intricacies of API design, focusing on five indispensable tips for creating exemplary APIs.
The Significance of Consistency and Predictability
At the heart of sound API design lie the principles of consistency and predictability. An API should boast a lucid and uniform structure that fosters developers' comprehension and utilization. All API operations must exhibit predictable behavior, with clearly delineated and well-defined responses for diverse inputs. This not only diminishes the likelihood of unforeseen errors but also streamlines the development process, empowering developers to seamlessly integrate the API into their projects.
To attain this coveted consistency, establishing a standardized naming convention for API operations and resources is paramount. This convention should extend across all endpoints, supplemented by meticulous documentation elucidating the inputs, outputs, and anticipated behavior of each operation. Furthermore, the API's design must prioritize intuitiveness and comprehensibility, achieved through the logical grouping of related operations and the provision of concise, unambiguous documentation.
# Sample endpoint naming convention
@app.route('/get_user_data', methods=['GET'])
def get_user_data():
# Logic to retrieve user data
return jsonify({'user_id': 1, 'name': 'John Doe'})
Designing for Flexibility and Scalability
API design must embody adaptability and scalability, primed to accommodate evolving software systems and burgeoning feature sets. It is imperative to foresee future requirements and weave them into the API's fabric, ensuring its capacity to seamlessly assimilate changes and expand alongside the software system's evolution.
A modular design approach emerges as a potent strategy in this pursuit. Breaking down the API into discrete, reusable components facilitates their facile combination and modification to meet evolving demands. This modular approach empowers the integration of new functionality and the refinement of existing components sans extensive alterations to the overarching API design.
# Modular API design with Flask-RESTful
from flask import Flask
from flask_restful import Api, Resource
app = Flask(__name__)
api = Api(app)
class Users(Resource):
def get(self, user_id):
# Logic to retrieve user data
return {'user_id': user_id, 'name': 'John Doe'}
class Orders(Resource):
def get(self, order_id):
# Logic to retrieve order data
return {'order_id': order_id, 'product': 'Sample Product'}
api.add_resource(Users, '/users/')
api.add_resource(Orders, '/orders/')
Harnessing the Power of HTTP Verbs
HTTP verbs constitute a pivotal facet of API design, serving as the language through which API operations communicate their intent. Proper employment of HTTP verbs is essential, as it reflects the nature of the operation being performed. The cardinal HTTP verbs encompass GET, POST, PUT, PATCH, and DELETE, each bearing a distinct purpose and deserving consistent usage throughout the API.
By adhering to the judicious use of HTTP verbs, developers can readily discern the purpose of each API operation, fostering a milieu of consistency and predictability. For instance, GET requests should be reserved for data retrieval, POST requests for resource creation, PUT and PATCH requests for resource updates, and DELETE requests for resource removal.
# Using HTTP verbs in Flask
from flask import Flask, request
app = Flask(__name__)
@app.route('/create_user', methods=['POST'])
def create_user():
# Logic to create a new user
data = request.json
# Process the user data
return jsonify({'message': 'User created successfully'})
@app.route('/update_user/', methods=['PUT'])
def update_user(user_id):
# Logic to update user data
data = request.json
# Process the updated data
return jsonify({'message': f'User {user_id} updated'})
@app.route('/delete_user/', methods=['DELETE'])
def delete_user(user_id):
# Logic to delete a user
# Delete the user with the specified ID
return jsonify({'message': f'User {user_id} deleted'})
Mastering the Art of Error Handling
Error handling assumes a pivotal role in API design, fortifying the API's capability to navigate unexpected scenarios adeptly. These scenarios encompass both client-side and server-side errors, spanning issues such as malformed or unauthorized requests.
To wield proficient error handling, the API must furnish cogent and informative error messages, complemented by judiciously assigned HTTP status codes that signify the error's nature. For instance, a 404 error code signifies the unavailability of a requested resource, while a 500 error code denotes server-side errors. Such meticulous error handling simplifies developers' comprehension of and response to errors, fostering an API that stands resilient and dependable.
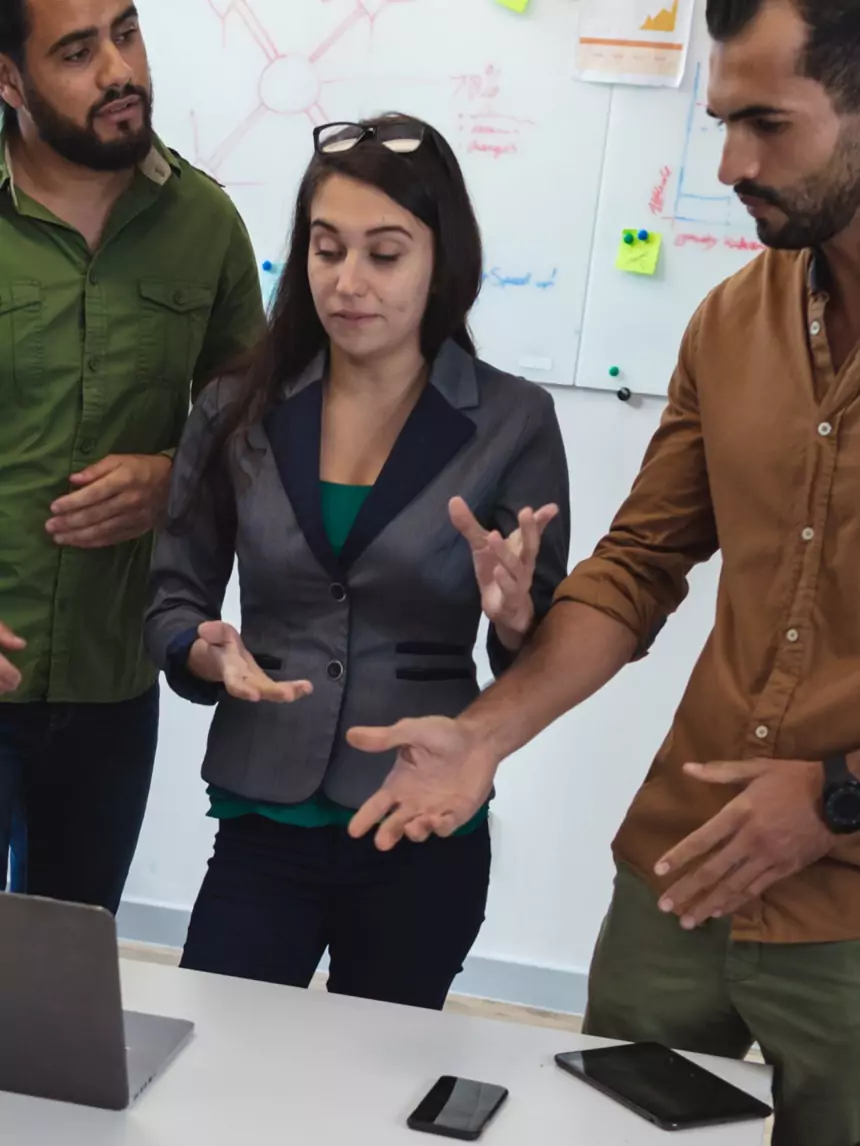
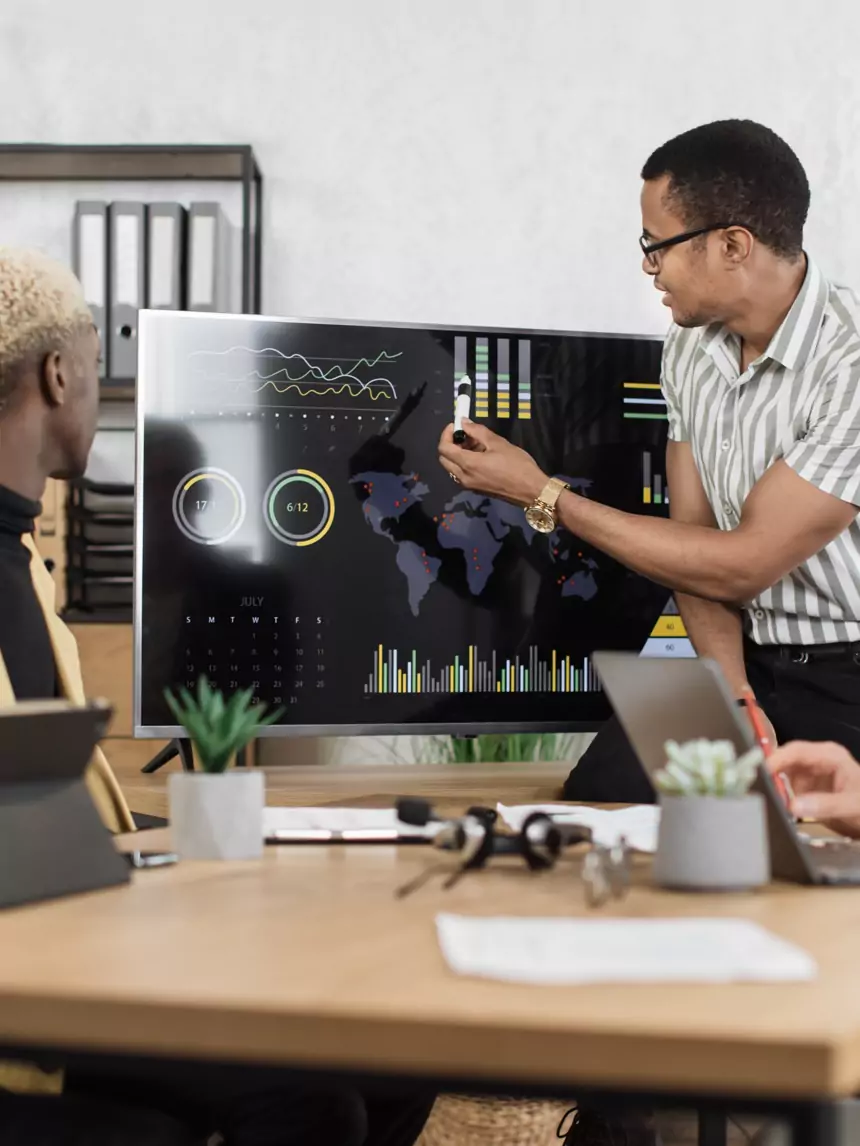
The Imperative of Comprehensive Documentation
Documentation stands as the lynchpin of API design, endowing developers with the knowledge requisite for efficacious API comprehension and utilization. Robust documentation must encompass clear and succinct elucidations of API operations, delineations of input and output formats, and insights into error handling mechanisms.
# Handling errors in Flask
from flask import Flask, jsonify, abort
app = Flask(__name__)
@app.route('/get_user/', methods=['GET'])
def get_user(user_id):
# Simulate a scenario where the user is not found
if user_id == 0:
abort(404, description="User not found")
return jsonify({'user_id': user_id, 'name': 'John Doe'})
@app.errorhandler(404)
def not_found_error(error):
return jsonify({'error': 'Not Found', 'message': error.description}), 404
API Design FAQ
What is API design, and why is it important?
API design refers to the process of creating a structured interface that allows different software components to communicate and share data seamlessly. It is crucial because it determines how effectively software systems can integrate and interact, impacting usability and performance.
What are the key principles of good API design?
Good API design hinges on principles like consistency, predictability, flexibility, scalability, proper use of HTTP verbs, meticulous error handling, and comprehensive documentation.
Why is consistency essential in API design?
Consistency ensures that the API has a clear and uniform structure, making it easier for developers to understand and use. It also minimizes the chances of unexpected errors.
How can I design an API to be flexible and scalable?
Employ a modular design approach, breaking the API into smaller, reusable components that can adapt to changing requirements. Anticipate future needs to ensure the API can evolve with the software system.
What is the significance of using HTTP verbs appropriately in API design?
Proper use of HTTP verbs indicates the purpose of each API operation, enhancing clarity and predictability. For example, GET requests retrieve data, while POST requests create new resources.
Why is error handling crucial in API design?
Effective error handling ensures the API can respond appropriately to unexpected situations, enhancing its robustness and reliability. It includes handling client-side and server-side errors with clear messages and HTTP status codes.
How can I provide comprehensive documentation for my API?
Comprehensive documentation should include clear explanations of API operations, input/output formats, and error handling. Use standardized naming conventions and offer concise and unambiguous documentation.
What are some common challenges in API design?
Common challenges include balancing simplicity with functionality, maintaining backward compatibility, and optimizing performance while adhering to design principles.
How does good API design impact software development projects?
Good API design accelerates development by providing a structured and consistent interface. It also promotes code reusability and simplifies integration, reducing development time and errors.
Can you recommend any tools or resources for API design?
Some popular tools for API design include Swagger, Postman, and API Blueprint. Additionally, resources like API design guidelines and best practices documents can be valuable references.
Conclusion
API design encapsulates the quintessence of software integration and interoperability. Its impact reverberates through modern software ecosystems, underpinning the creation of applications that seamlessly communicate and collaborate. By hewing to the tenets of consistency and predictability, embracing flexibility and scalability, harnessing HTTP verbs judiciously, mastering error handling, and furnishing comprehensive documentation, API designers equip themselves to craft interfaces that transcend mere functionality, culminating in harmonious software landscapes where disparate components converge in concert.
Tyrone Showers