Co-Founder Taliferro
Software Development
Software development drives innovation and efficiency. At Taliferro, we build high-performing development teams focused on delivering quality products. Here's how we do it with Agile methodology and other key strategies.
Agile Methodology: Adapting to Change
Agile drives adaptability and continuous improvement. With Scrum or Kanban, teams stay flexible, responding to shifting requirements and client feedback, ensuring consistent delivery.
# Example of a user story in Agile using Scrum
class UserStory:
def __init__(self, title, description):
self.title = title
self.description = description
self.status = "To Do"
def move_to_next_status(self):
if self.status == "To Do":
self.status = "In Progress"
elif self.status == "In Progress":
self.status = "Done"
# Creating a user story
story = UserStory("Implement User Authentication", "As a user, I want to be able to log in to my account.")
# Moving the user story through Scrum stages
story.move_to_next_status() # Moves from "To Do" to "In Progress"
story.move_to_next_status() # Moves from "In Progress" to "Done"
Building Cross-Functional Teams
Software development thrives on collaboration. Taliferro builds cross-functional teams with developers, testers, designers, and product managers. The result? Comprehensive solutions that meet every stakeholder’s needs.
CI/CD: Automating for Efficiency
Automation keeps projects moving. With CI/CD pipelines, teams reduce errors, speed up feature releases, and deliver rapid updates to clients.
# Example of a simple CI/CD pipeline using YAML (for demonstration purposes)
stages:
- build:
script:
- echo "Building the software..."
- test:
script:
- echo "Running tests..."
- deploy:
script:
- echo "Deploying to production..."
Code Reviews and Pair Programming
Collaboration through code reviews and pair programming ensures quality. These practices spot issues early and promote shared understanding of the codebase.
Test-Driven Development (TDD)
TDD ensures precise, maintainable code. Writing tests first leads to thorough testing and fewer bugs. This process builds reliable functionality into every line of code.
Documentation and Knowledge Sharing
Documentation maintains consistency. By documenting code and decisions, teams streamline development and ensure clear understanding across the board.
Training and Development
We invest in talent. Through ongoing training and conferences, our teams stay at the forefront of industry trends, delivering cutting-edge solutions.
Performance Monitoring and Optimization
Efficiency matters. Monitoring performance helps identify bottlenecks and optimize code for high-performing software.
Client Communication
Clear communication keeps projects aligned with client goals. Frequent updates ensure quick responses to changing needs.
Security
Security is non-negotiable. Regular audits, encryption, and safe coding practices protect software from threats.
Quality Assurance (QA)
QA works alongside developers to ensure issues are caught early. Rigorous testing maintains reliability and ensures client satisfaction.
Scalable Infrastructure
Scaling is key. With cloud infrastructure and microservices architecture, we build scalable solutions ready for growth.
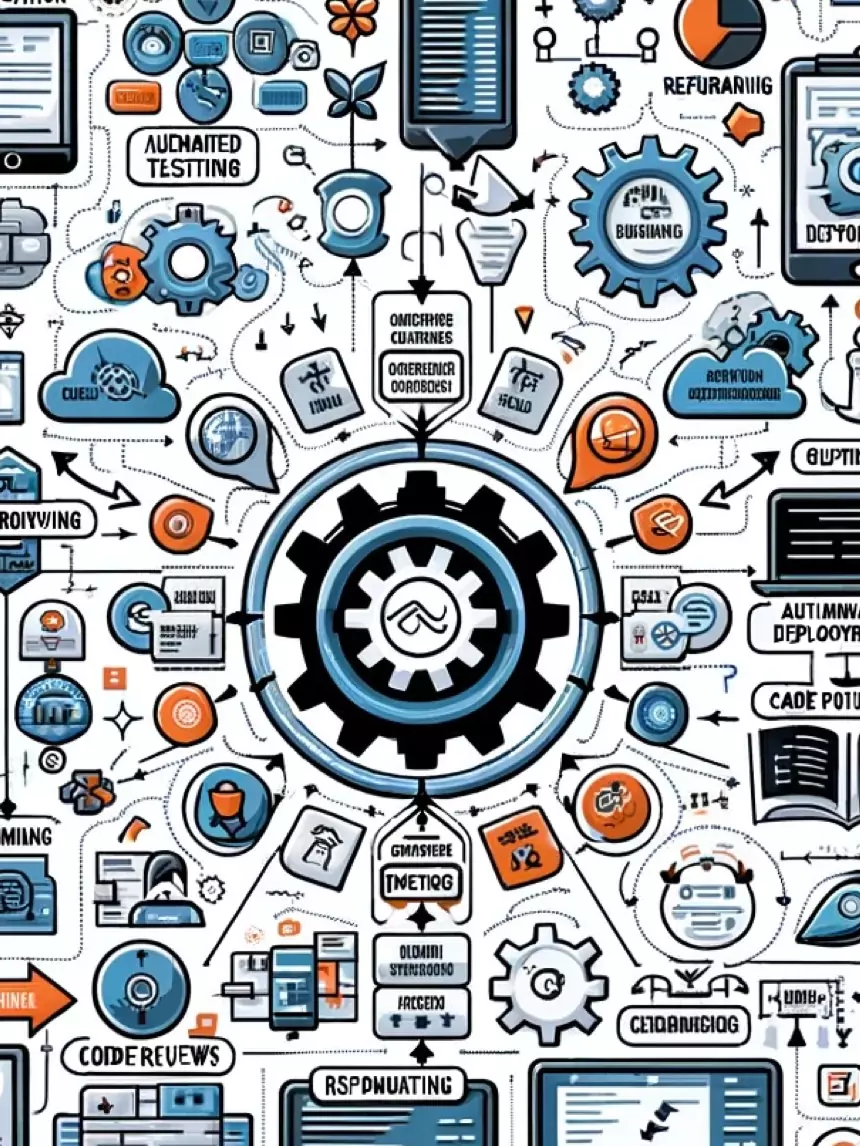
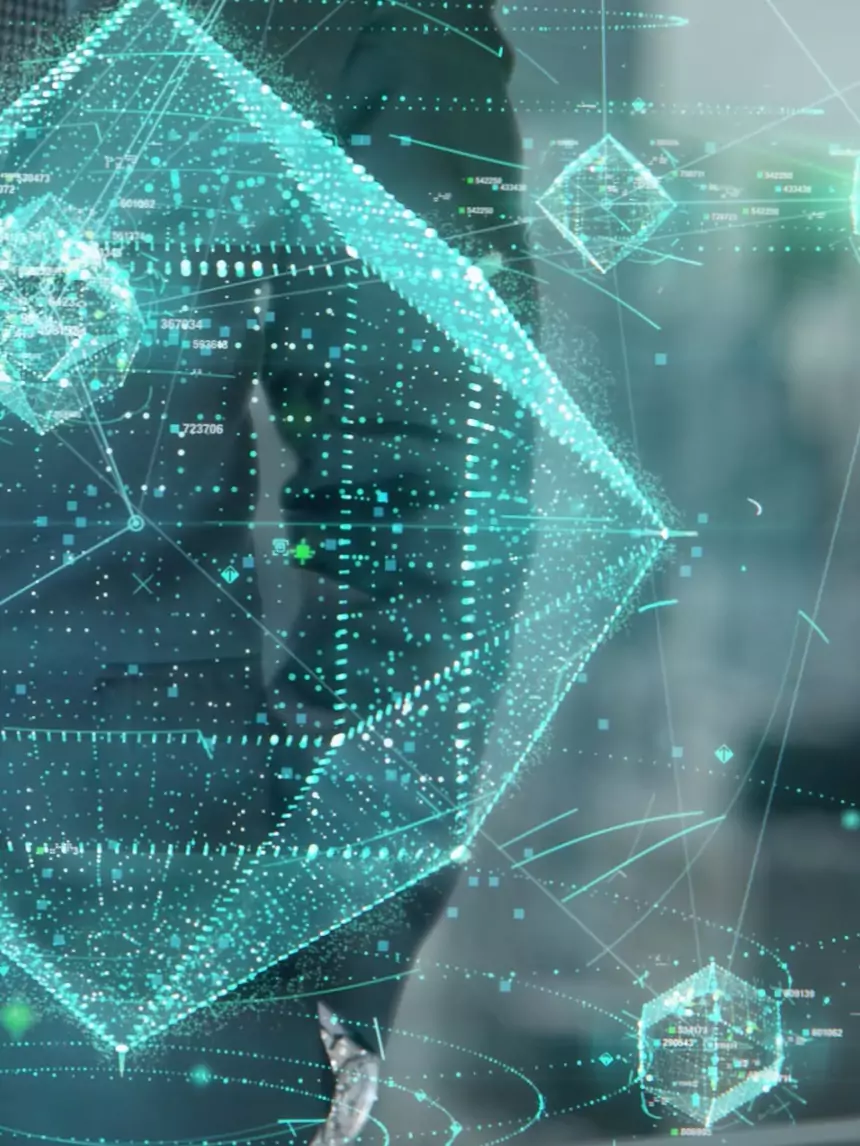
Time Management
Effective time management ensures projects stay on schedule. Task breakdowns, progress tracking, and resource allocation are essential.
Risk Management
Proactive risk management minimizes issues. Identifying risks early leads to smooth project execution.
Client Feedback
Client feedback refines processes. Continuous input helps us enhance products and improve experiences.
Reusable Code and Components
Efficiency through reusable code. Pre-built modules speed up development and maintain quality.
Innovation and Experimentation
Innovation drives success. Encouraging experimentation keeps teams ahead of the curve with the latest technology and techniques.
Adaptability
Adaptability ensures success. Teams stay flexible, adjusting to market demands and client needs as they arise.
# Example of a simple test-driven development scenario in Python
def add_numbers(num1, num2):
return num1 + num2
# Writing a test case
def test_add_numbers():
assert add_numbers(2, 3) == 5
assert add_numbers(-1, 1) == 0
assert add_numbers(0, 0) == 0
# Running the test case
test_add_numbers()
Leadership and Vision
Strong leadership and clear vision guide success. Taliferro builds leadership that sets ambitious goals and provides the resources to meet them.
Company Culture
A culture of excellence drives everything. Collaboration, quality, and continuous improvement remain central to our approach.
Conclusion
With Taliferro’s strategies, your team can deliver high-quality, innovative software. Our commitment to excellence keeps you ahead in software development, ready to face new challenges and opportunities.